JavaScript
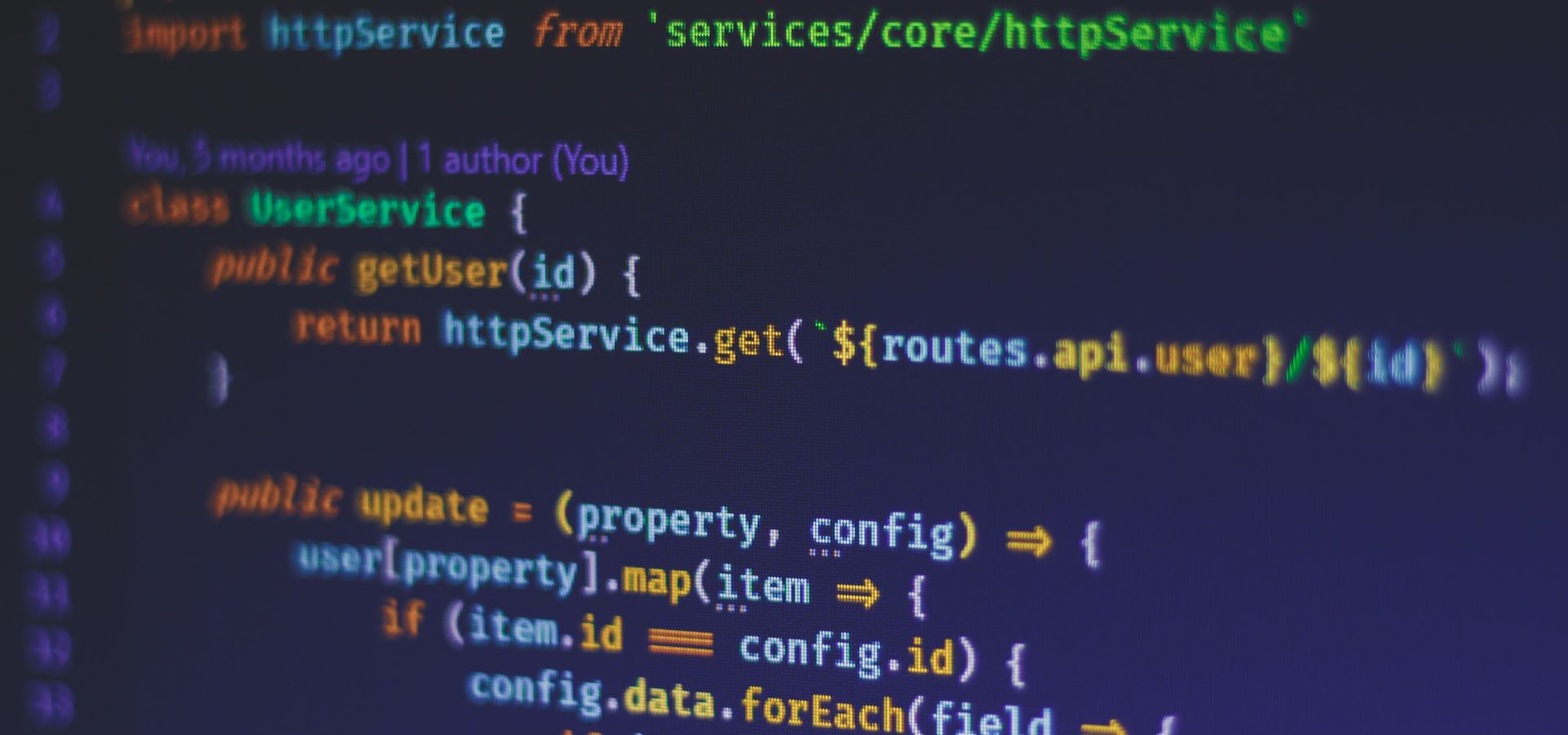
JavaScript, often abbreviated as JS, is a dynamic and versatile programming language that powers interactivity and functionality on the web. It is a fundamental technology for web development, allowing developers to create dynamic, responsive, and engaging user experiences.
javascript/* Using variable inside strings */
const string = `This ${someVariable} is inside a string.`;
/* For-loop */
for(let i = 0; i < someArray.length; i++) {
// Do stuff here while i is less than length of someArray
}
/* Do-while loop */
let result = '';
let i = 0;
do {
i = i + 1;
result = result + i;
} while (i < 5);
/* Ternary operator */
const someNumber = Get number from somewhere
let state1 = true;
let state2 = false;
let result = someNumber === 10 ? state1 : state2;
// result outputs true or false
/* Intersection observer */
const container = document.getElementById("someElementId");
const observer = new IntersectionObserver(elements => {
elements.forEach(el => {
if(el.isIntersecting) {
// Do stuff here when element is intersecting
// Unobserve if it should be triggered only once
observer.unobserve(container);
}
}
)
},
{
// Options
threshold: 0.5
}
);
// Initiate observation
observer.observe(container);
/* Modal width dialog element */
const showButton = document.getElementById('showDialog');
const closeButton = document.getElementById('cancelBtn');
const dialog = document.getElementById('dialog');
// "Show the dialog" button opens the <dialog> modally
showButton.addEventListener('click', () => {
// Enabled background interaction
//dialog.show();
// Disabled background interaction
dialog.showModal();
});
// "Close the dialog" button closes the <dialog>
closeButton.addEventListener('click', () => {
dialog.close();
});
dialog.addEventListener("click", (event) => {
const rect = dialog.getBoundingClientRect();
if (event.clientY < rect.top || event.clientY > rect.bottom || event.clientX < rect.left || event.clientX > rect.right) {
dialog.close();
}
});